1. (17%) Answer each of the following questions.
(A) Given an infix expression (a+b)/c*(d-e), please write down the corresponding prefix and postfix expressions.
(B) Given the following binary tree, which corresponds to a Left Child-Right Sibling representation of a general tree. Please draw the input general tree.
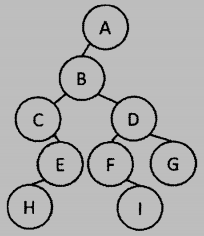
(C) Given the following binary search tree (BST), please draw ALL possible tree(s) after deleting the node with key 48.
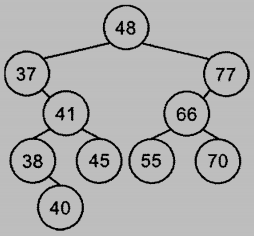
(D) Assume the pre-order and in-order traversal of a binary tree are ABDECFHGI and EDBAHFCGI, respectively. Please draw the tree, and explain whether or not the tree is unique.
(E) Assume the pre-order and post-order traversal of a binary tree are ABDECFHGI and EDBHFIGCA, respectively. Please draw the tree, and explain whether or not the tree is unique.
(F) A clocked tree is a binary tree in which each node ni is associated with a non-negative delay, delay(ni). The path delay from a root to a node is defined as the summation of delay of all nodes along the path. The longestDelay is defined as the longest path delay among all root-to-leaf paths. Please base on the class definition show on the right to write pseudo codes of function: void longestDelay(node *root, int AccDelay); that computes and stores the longest path delay in the variable “MAX”.
Class definition
class node {
int delay;
treenode *lchild;
treenode *rchild;
}
int MAX;
參考解答:
(A) Prefix: */+abc-de Postfix: ab+c/de-*
(B)
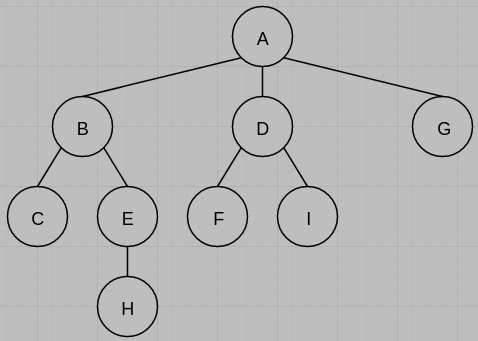
(C)
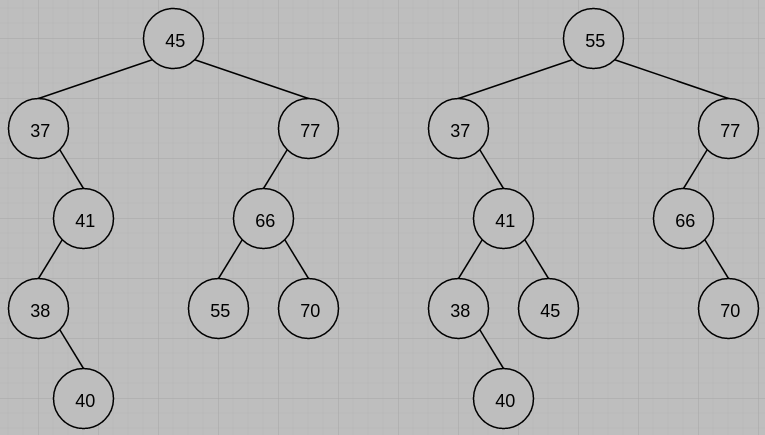
(D)
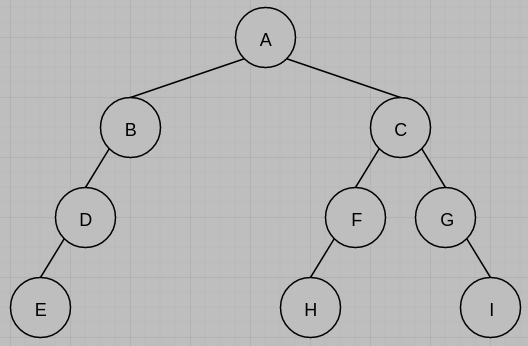
(E)
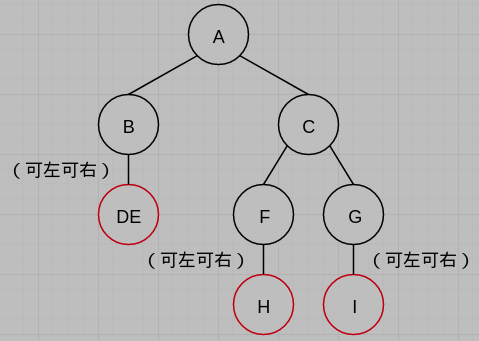
The tree is not unique. (There are 8 binary trees)
(F)
void longestDelay(node *root, int AccDelay)
{
if (root == null) return;
AccDelay += root -> delay;
if (root -> lchild)
longestDelay(root -> lchild, AccDelay);
if (root -> rchild)
longestDelay(root -> rchild, AccDelay);
if (root -> lchild == null && root -> rchild == null)
{
if (MAX < AccDelay)
MAX = AccDelay;
}
}
2. (17%) Answer each of the following questions.
(A) Give a definition for the reflexive transitive closure matrix A∗ of a directed graph G.
(B) What does the following function compute?
void Function1(int cost[][MAX_VERTICES], int distance[][MAX_VERTICES], int n)
{
/* cost is the adjacency matrix of some directed graph */
int i, j, k;
for (i = 0; i < n; i++)
for (j = 0; j < n; j++)
distance[i][j] = cost[i][j];
for (k = 0; k < n; k++)
for (i = 0; i < n; i++)
for (j = 0; j < n; j++)
if (distance[i][k] + distance[k][j] < distance[i][j])
distance[i][j] = distance[i][k] + distance[k][j];
}
(C) Prove or disprove that a graph is bipartite only if it contains no cycles of odd length.
(D) What is a topological sort (or topological ordering) of a directed acyclic graph?
(E) Describe a linear time O(|V|+|E|) algorithm for the topological sort of a graph G=(V,E) using the depth first search implementation.
參考解答:
(A)
{A∗[i,i]=1A∗[i,j] if there exist path from i to j, set to 1, else set to 0.
(B) All pairs shortest path
(C) 若bipartite有odd length cycle, 將vertex分成v1,v2兩種。假設起點為u1∈v1, 則終點u2∈v2 (因odd length), 此時cycle不存在。Contradiction found.
(D) An order we use in AOV or AOE network to find whether a job needs to be done before another job.
(E) We can use DFS and then output the nodes in a descendant order from the finish time, that will be a topological sort.
3. (17%) Answer each of the following questions.
(A) Let x and y be integers and m be a positive integer, then x is congruent to y modulo m if (x-y) is divisible by m, we denote this fact as x≡ymodm. Fermat’s Little Theorem states that if p is prime and z is an integer not divisible by p then zp−1≡1modp.
Use Fermat’s Little Theorem to compute 3302mod5,3302mod7,3302mod11, respectively.
(B) Use the results in (a) and Chinese Remainder Theorem to find 3302mod385. (Note that 385=5⋅7⋅11)
(C) Solve the simultaneous recurrent relations
an=3an−1+2bn−1bn=an−1+2bn−1with a0=2,b0=1.
參考解答:
(A) 4, 2, 9.
(B) 9
(C) an=2⋅4n,bn=4n,n≥0.
4. (17%) Answer each of the following questions.
(A) How many different strings can be made by reordering the letters of the word SUCCESS?
(B) Assume that a sequence {an} satisfies the recurrence relation an=8an−1+10n−1 and the initial condition a1=9. Use generating functions to find an explicit formula for an.
(C) Consider the following relations on {1,2,3,4},
- R1 = {(1,1),(1,2),(2,1),(2,2),(3,4),(4,1),(4,4)},
- R2 = {(1,1),(1,2),(2,1)},
- R3 = {(1,1),(1,2),(1,4),(2,1),(2,2),(3,3),(4,1),(4,4)},
- R4 = {(2,1),(3,1),(3,2),(4,1),(4,2),(4,3)},
- R5 = {(1,1),(1,2),(1,3),(1,4),(2,2),(2,3),(2,4),(3,3),(3,4),(4,4)},
- R6 = {(3,4)}.
Which of these relations are symmetric? anti-symmetric? transitive?
參考解答:
(A) 7!3!2!
(B) an=12(8n+10n),n≥1.
(C) Symmetric: R2,R3. Anti-symmetric: R4,R5,R6. Transitive: R4,R5,R6.
5. (17%) Answer each of the following questions.
(A) Let u and v be two n bit numbers, where we assume that n is a power of 2 for simplicity. Clearly, multiplying u and v straightforwardly requires O(n2) steps.
(a) Please design a divide-and-conquer approach to compute the product of u and v in O(nlog23) time.
(b) Explain why the time complexity of your divide-and-conquer approch is O(nlog23).
(B) Determine whether the following statements are correct or not and also justify your answers. No points are given for answers without justification.
(a) The Cook’s theorem states that if the SAT (Satisfiability) problem is in NP, then P = NP.
(b) If we want to prove that a decision problem X is NP-complete, it is enough to reduce X to the SAT problem.
(c) For an NP-complete problem, we need to take exponential time to solve this problem for all kinds of inputs.
參考解答:
(A)
(a) Let u=u1u2…un and v=v1v2…vn→uL=u1u2…un2−1,uR=un2un2+1…un,vL=v1v2…vn2−1, and vR=vn2vn2+1…vn.
Step 1: A=uL×vL
Step 2: B=uR×vR
Step 3: C=uL×vR+uR×vL
Step 4: Put A, B, and C in the right position and add them to get the result.
(b) Let T(n) be the time to compute n bits×n bits, then T(n)=3T(n2)+O(n), so the time complexity is O(nlg3).
(B) (a) F (b) F (c) F
6. (15%) Answer each of the following questions.
(A) The following algorithm takes an n by n matrix A(1:n,1:n) as input, does LU-decomposition and stores the results in the same array A(1:n,1:n), where L is a unit lower triangular matrix (the diagonal elements are all 1s, and the elements above the diagonals are all 0s) and U is an upper triangular matrix (the elements below the diagonals are all 0s). We further assume that a given matrix can be LU-decomposed in our case.
for i = 1,2,...,n-1
for k = i+1,...,n
t = -A(k,i)/A(i,i);
for j = i+1,...,n
A(k,j) = A(k,j) + t*A(i,j);
endfor
A(k,i) = -t;
endfor
endfor
Given a 3 by 3 matrix as stated below as an input
A=[11−124−1−1−33]
What are the contents of A after implementing the algorithm? (Show integer parts only).
(B) Let n≥m be positive integers and let r be a nonnegative integers. The following algorithm will compute gcd(n,m), the greatest common divisor of n and m.
while (m > 0) do
{r = n mod m; n = m; m = r;}
Return n
Given n = 13923 and m = 13056 as input, find the output of n.
參考解答:
(A)
[11−1221−1−13]
(B) 51
試題(pdf):連結
有任何問題,或想要完整解釋的,歡迎在下方留言唷!
aaa
嗨,第一題(f)小題的程式碼是不是有誤?因為第7行if statement的條件是「只有」當root的兩個child為null,若root還有左右child,recursion就無法繼續拜訪下去了,所以是否將裡面12和15行兩個判斷式和其中的遞迴式移出第7行的if statement並放在AccDelay+=root->value下方,讓只有當current node為leaf時才更新MAX,可以討論看看嗎?
mt
對誒~原本的的確有bug!像你這樣改就可以了喔~感謝!
leo
想問
第四小題的B小題
原先是想說是
An=An-1 +10^(n-1)
因為題目說初始條件是A1
是否代表A0不存在
mt
我想這題他一開始給a1而且也沒有用到a0所以自然就不會去在意a0是什麼了~